Hi,
What kits are you using (mBot, Starter, Ranger)?
I’m working on the second volume in my mBot series that focuses on Arduino programming (in my not-so-copious spare time). The first thing I’d observe is that the version of Arduino that ships with mBlock 3.4.11 is a bit out-of-date. It works, but the latest version has a lot of improvements. If you are focusing on Arduino programming, then you may want to consider installing the current version of the Arduino programming environment and installing the Makeblock library into the sketchbook. Just my opinion and your mileage may vary. 
The mBlock environment lets you see what Arduino code is generated by the blocks by selecting the Arduino mode from the Edit menu. A lot of folks start there so that they can see working examples. For example, the following code blocks:
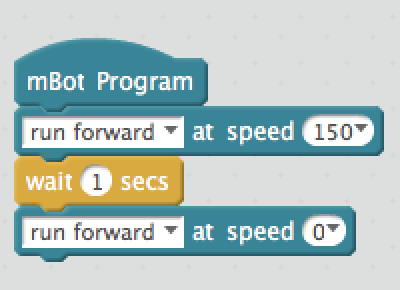
generates the following code (seen in the Arduino mode editor pane):
#include <Arduino.h>
#include <Wire.h>
#include <SoftwareSerial.h>
#include <MeMCore.h>
MeDCMotor motor_9(9);
MeDCMotor motor_10(10);
void move(int direction, int speed)
{
int leftSpeed = 0;
int rightSpeed = 0;
if(direction == 1){
leftSpeed = speed;
rightSpeed = speed;
}else if(direction == 2){
leftSpeed = -speed;
rightSpeed = -speed;
}else if(direction == 3){
leftSpeed = -speed;
rightSpeed = speed;
}else if(direction == 4){
leftSpeed = speed;
rightSpeed = -speed;
}
motor_9.run((9)==M1?-(leftSpeed):(leftSpeed));
motor_10.run((10)==M1?-(rightSpeed):(rightSpeed));
}
double angle_rad = PI/180.0;
double angle_deg = 180.0/PI;
void setup(){
move(1,150);
_delay(1);
move(1,0);
}
void loop(){
_loop();
}
void _delay(float seconds){
long endTime = millis() + seconds * 1000;
while(millis() < endTime)_loop();
}
void _loop(){
}
There is a lot of boilerplate code which it typical of a code generator. The code could be simplified into:
#include <MeMCore.h>
// declare motor objects
MeDCMotor left_motor(9);
MeDCMotor right_motor(10);
// move forward method
void move_forward(int speed)
{
// The motors must spin in opposite directions
// to move the robot in a forward motion
left_motor.run(-speed);
right_motor.run(speed);
}
// setup method - runs once
void setup(){
// move forware at speed of 150
move_forward(150);
// wait 1 second
_delay(1);
// stop moving (speed 0)
move_forward(0);
}
// wait until the time has expired
void _delay(float seconds) {
// The delay() method is a blocking call which is fine for
// this example. A blocking call makes everything else wait
// until it completes. This will not affect the motors because
// they have been told to run at a certain speed until
// further notice.
//
// the code generate by mBlock uses a non-blocking call so
// that any operations in the loop_() method (not shown in
// this snippet) will continue to be executed.
delay(seconds * 1000);
}
// loop method runs continuously after setup method completes
void loop(){
}
Hope this helps. 
Chuck